使用代码
"""
@FileName: Test.py
@Description: Implement Test
@Author: Ryuk
@CreateDate: 2020/12/08
@LastEditTime: 2020/12/08
@LastEditors: Please set LastEditors
@Version: v0.1
"""
from td_psola import *
import librosa
import soundfile as sf
import matplotlib.pyplot as plt
x, fs = librosa.load("./test.wav", sr=8000)
pitch_scale = 1.5
time_scale = 1.5
y = Processing(x, fs, pitch_scale, time_scale, cutoff_freq=500)
sf.write("./out.wav", y, fs)
fig=plt.figure()
ax=fig.add_subplot(2, 1, 1)
ax.plot(x)
ax=fig.add_subplot(2, 1, 2)
ax.plot(y)
plt.savefig("q.png")
if __name__ == __main__:
pass
实际代码
"""
@FileName: Algorithm.py
@Description: Implement Algorithm
@Author: Ryuk
@CreateDate: 2020/12/07
@LastEditTime: 2020/12/07
@LastEditors: Please set LastEditors
@Version: v0.1
"""
import numpy as np
from scipy import signal
def Processing(x, fs, pitch_scale, time_scale, cutoff_freq=500):
# normalize
x = x - np.mean(x)
x = x / np.max(np.abs(x))
#x = LowPassFilter(x, fs, cutoff_freq)
pitch = PitchEstimator(x, fs)
output = PitchMark(x, pitch, fs, pitch_scale, time_scale)
return output
def LowPassFilter(x, fs, cutoff_freq):
if cutoff_freq == 0:
return x
else:
factor = np.exp(-1 / (fs / cutoff_freq))
y = signal.filtfilt([1 - factor], [1, -factor], x)
return y
def PitchEstimator(x, fs):
frame_length = round(fs * 0.03)
frame_shift = round(fs * 0.01)
length = len(x)
frame_num = int(np.floor((length - frame_length)/ frame_shift)) + 2
frame_pitch = np.zeros(frame_num + 2)
frame_range = np.arange(0, frame_length)
for count in range(1, frame_num):
frame = x[frame_range]
frame_pitch[count] = PitchDetection(frame, fs)
frame_range += frame_shift
frame_pitch = signal.medfilt(frame_pitch, 5)
pitch = np.zeros(length)
for i in range(length):
index = int(np.floor((i + 1) / frame_shift))
pitch[i] = frame_pitch[index]
return pitch
def CenterClipping(x, clip_rate):
max_amplitude = np.max(np.abs(x))
clip_level = max_amplitude * clip_rate
positive_index = np.where(x > clip_level)
negative_index = np.where(x < -clip_level)
clipped_data = np.zeros(len(x))
clipped_data[positive_index] = x[positive_index] - clip_level
clipped_data[negative_index] = x[negative_index] + clip_level
return clipped_data
def AutoCorrelation(x, lags):
N = len(x)
auto_corr = np.correlate(x, x, mode = full)
assert N >= lags - 1
auto_corr = auto_corr[N - lags - 1 : N + lags]
auto_corr = auto_corr / np.max(auto_corr)
return auto_corr
def IsPeak(index, low, high, x):
if index == low or index == high:
return False
if x[index] < x[index-1] or x[index] < x[index+1]:
return False
return True
def PitchDetection(x, fs):
min_lag = round(fs / 500)
max_lag = round(fs / 70)
x = CenterClipping(x, 0.3)
auto_corr = AutoCorrelation(x, max_lag)
auto_corr = auto_corr[max_lag: 2 * max_lag]
search_range = auto_corr[min_lag - 1:max_lag]
max_corr
文章版权归作者所有,未经允许请勿转载,若此文章存在违规行为,您可以联系管理员删除。
转载请注明本文地址:https://www.ucloud.cn/yun/124400.html
相关文章
-
如何反转CSS中的贝塞尔曲线
摘要:我们并不需要知道贝塞尔曲线背后的所有数学知识。我们可以使用相同的并设置并反转贝塞尔曲线,这样就实现了在正反两个方向上使用同一个的效果。我们来看看如何计算反向的贝塞尔曲线。 首先来看一看我之前写的一个CSS轮播动画效果,为了让切换时动画的过渡更加的平滑我在animation-timing-function属性中并没有使用CSS提供的各种关键词,而使用了cubic-bezier(贝塞尔)函...
-
如何反转CSS中的贝塞尔曲线
摘要:我们并不需要知道贝塞尔曲线背后的所有数学知识。我们可以使用相同的并设置并反转贝塞尔曲线,这样就实现了在正反两个方向上使用同一个的效果。我们来看看如何计算反向的贝塞尔曲线。 首先来看一看我之前写的一个CSS轮播动画效果,为了让切换时动画的过渡更加的平滑我在animation-timing-function属性中并没有使用CSS提供的各种关键词,而使用了cubic-bezier(贝塞尔)函...
-
如何反转CSS中的贝塞尔曲线
摘要:我们并不需要知道贝塞尔曲线背后的所有数学知识。我们可以使用相同的并设置并反转贝塞尔曲线,这样就实现了在正反两个方向上使用同一个的效果。我们来看看如何计算反向的贝塞尔曲线。 首先来看一看我之前写的一个CSS轮播动画效果,为了让切换时动画的过渡更加的平滑我在animation-timing-function属性中并没有使用CSS提供的各种关键词,而使用了cubic-bezier(贝塞尔)函...
-
JavaScript基本运动封装函数(1)
摘要:为回调函数等于已过时间初始值距离总时间匀速加速曲线减速曲线加速减速曲线加加速曲线减减速曲线加加速减减速曲线正弦衰减曲线弹动渐入正弦增强曲线弹动渐出回退加速回退渐入回缩的距离弹球减振弹球渐出 function doMove(obj, json, time, fx, fn) //fn为回调函数 { clearInterval(obj.iTimer); var fx = fx || li...
发表评论
0条评论
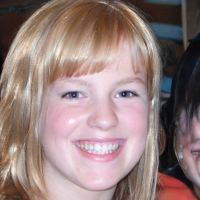
番茄西红柿
男|高级讲师
TA的文章
阅读更多tensor
阅读 435·2023-04-25 19:43
Windows 下安装 XGBoost
阅读 3627·2021-11-30 14:52
Hadoop 2.6.0 启动问题 lib/native/libhadoop.so which mi
阅读 3420·2021-11-30 14:52
VmShell:黑五美国VPS,免费先开通测试,满意后付款!支持tiktok美区
阅读 3527·2021-11-29 11:00
百度智能云:云产品特惠福利,1核2G轻量应用服务器仅48元/年
阅读 3496·2021-11-29 11:00
Linux系统和宝塔面板如何启用禁ping功能?
阅读 3525·2021-11-29 11:00
301重定向怎么做?301重定向设置方法有几种
阅读 3264·2021-11-29 11:00
wordpress网站重定向次数过多的解决方法
阅读 5425·2021-11-29 11:00